Building a Login Flow with .NET MAUI
Let's build a Login Flow with .NET MAUI with Shell.
Authentication in any mobile app is very common. Lets get started with this. Its obvious that it should ask for login only if it isn't authenticated. We will check for authentication , if not there we will move to Login page if login is success we will move to the Home page. For this example we will override the backbutton pressed event to quit the application but you can customize accordingly as per your need.
For this post I am using a simple authentication but you can use JWT or any method you want.
Here is the example of the login flow:
All the pages that has to be used needs to be registered with the Shell.
If you are a bit familier with the Shell navigation the first content page is the one which is displayed after startup. So we need to structure the shell accordingly in order.
The pages we are using here for the example:
- LoadingPage
- LoginPage
- HomePage
- SettingsPage
Here is the AppShell.xaml
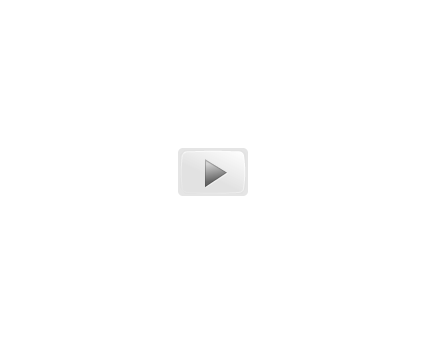
So we can override the OnNavigatedTo method to check if the app is authenticated not not. Here is the code which can be written in the page or in the view model.
The back button pressed event
The Login page:
When the app isn't authenticated it goes to the LoginPage. I haven't placed any validation for the controls but it can be put. I have hardcoded the username and password to authenticate for simulating the login flow.
Here is the login page with one button and two entry controls for Username and Password.
Here is the click event:
If the username and password is correct the we can navigate to HomePage.
The Home Page:
The SettingsPage:
In the settings page we can implement the logout logic. In the log out button clicked we can write like this.
Conclusion:
Its a very small example for building a login flow in .NET MAUI. You can check out.the repository in GitHub.
Hi, I am trying to implement your LoginFlow example in a Maui App I am developing. I undertand the code and logic and most is working. However I do have one problem with the line:
ReplyDeleteawait Shell.Current.GoToAsync("///home"); in particular the three forward slashes.
When I run your code I do not get an error.
However when I run the same code in my app I get the error:
System.Exception: 'Global routes currently cannot be the only page on the stack, so absolute routing to global routes is not supported. For now, just navigate to: /mainPage'
I want my MainPage to be the Shell root so I need to be able to use ///mainPage.
An internet search acknowledges the issue and suggests work arounds but does not give a fix.
My question is how does /// work in your code without raising an exception?
Many thanks for your help.